Latest news about Bitcoin and all cryptocurrencies. Your daily crypto news habit.
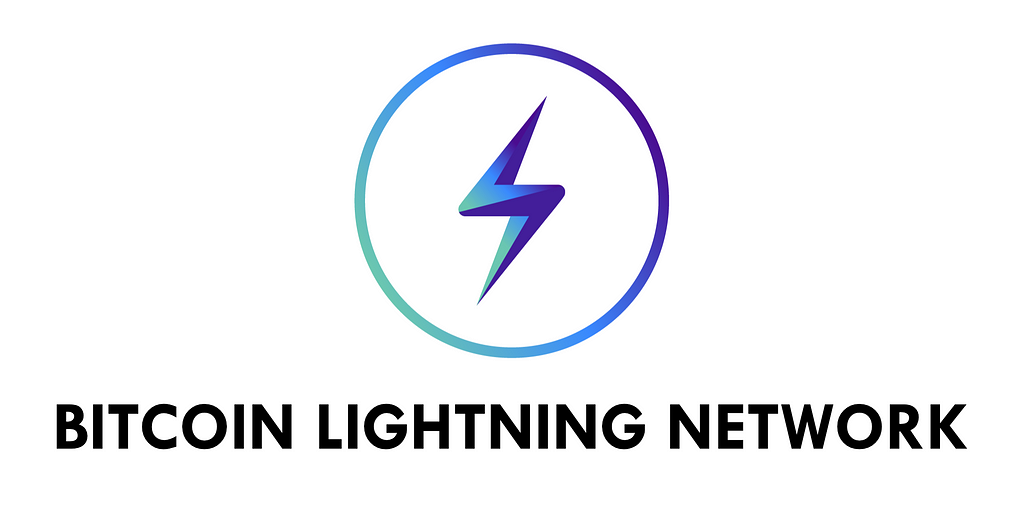
This article is meant for Bitcoin LND beginners.
Bitcoin’s Lightning Network is in short “Scalable, Instant Bitcoin/Blockchain Transactions”. It is the concept of having “payment channels” that users can leverage “off-chain”(meaning not on the Bitcoin blockchain) to send nearly instant and low cost transactions. Here is a link to a more detailed description of it: http://lightning.network/how-it-works/.
The Lightning Network Daemon (LND) is the “complete implementation of a Lightning Network node”. All you really need to know from this is that it allows us to set up a lightning network on our own computers fairly easily. Their Github: https://github.com/lightningnetwork/lnd
I personally think the best way to learn things is by implementing them into some sort of project. However, it turned out setting up the LND in order to be able to program with it was the toughest part. So here we go!
Before you get started…
Who is this guide aimed at? Like I said at the beginning of this article, this is meant for LND beginners and you don’t even need to be that proficient at Go to follow either. I am using a Mac to do everything but hopefully you have enough knowledge to do the same things with your OS. We will be using the REGTEST bitcoin server for this.
1st thing: Install Go <- There is a great tutorial in that link
2nd thing: Install Bitcoind, personally I suggest you just run:
brew install bitcoin
because you NEED to have ZeroMQ installed with bitcoind in order for LND to work and the brew install will do almost everything for you. The reason I say almost is because we still need to add a config file. Beyond that, here is a tutorial on how to install bitcoind on mac (without ZeroMQ), but it still has great documentation on what to do after it is installed.
Once Bitcoind is installed, we can add the config file with these 3 commands,
For mac:
mkdir -p "/Users/${USER}/Library/Application Support/Bitcoin"
touch "/Users/${USER}/Library/Application Support/Bitcoin/bitcoin.conf"
chmod 600 "/Users/${USER}/Library/Application Support/Bitcoin/bitcoin.conf
command 1: Creates a folder named Bitcoin
command 2: Creates a file named bitcoin.conf
command 3: Changes the permissions of the file to allow the owner to read/write
bitcoin.conf file contents:
rpcuser=bitcoinrpcrpcpassword=some_password_goes_heretestnet=0regtest=1server=1daemon=1zmqpubrawblock=tcp://127.0.0.1:28332zmqpubrawtx=tcp://127.0.0.1:28333
This should be added to your bitcoin.conf file.
At this point you should simply be able to type:
bitcoind
Into your terminal and this should appear:
Bitcoin server starting
You now have a bitcoin REGTEST server running in the background.
*NOTE: Regtest means that you will not download the entire Bitcoin blockchain, but rather you can manually create blocks and create bitcoin. Allowing us to test without the large amount of space required to hold the bitcoin mainnet blockchain.
To STOP the bitcoin server run:
bitcoin-cli stop
3rd thing: Install LND
Go here for their official installation guide, it is very easy to install. Once installed you should have 2 items added to your go/bin folder:
- lncli
- lnd
Once LND is installed, we can add the config file with these 3 commands,
For mac:
mkdir -p "/Users/${USER}/Library/Application Support/Lnd"
touch "/Users/${USER}/Library/Application Support/Lnd/Lnd.conf"
chmod 600 "/Users/${USER}/Library/Application Support/Lnd/Lnd.conf
lnd.conf file contents:
bitcoin.regtest=1bitcoin.active=1bitcoin.node=bitcoinddebuglevel=debug
This should be added to your lnd.conf file.
Most likely you will not simply be able to type “lnd” into you terminal and have the server start. In order to do that you most likely want to create an alias in your /.bash_profile.
Open .bash_profile with:
open ~/.bash_profile
and add these lines to the end of the file:
# LND commands from terminalalias lnd=$HOME/go/bin/lndalias lncli=$HOME/go/bin/lncli
these commands are meant to point to your go/bin/lnd and go/bin/lncli binaries, so if your file structure is different then this, you must edit the path.
Finally you should be able to start the LND server by simply typing:
lnd
You should get text like this when it runs successfully:
Waiting for wallet encryption password. Use `lncli create` to create a wallet, `lncli unlock` to unlock an existing wallet, or `lncli changepassword` to change the password of an existing wallet and unlock it.
To create a wallet encryption password, in a new terminal type:
lncli create
Note down the password you create and do not add a mnemonic or a passphrase.
All together now.
In terminal 1, start the bitcoin server and the lnd server:
bitcoind
lnd
In terminal 2, unlock the lnd wallet:
lncli unlock
Step 1: GRPC setup
LND uses the LND gRPC API for their CLI. You may have heard of REST API’s, well gRPC is an alternative to that. Currently the LND API docs only show Javascript and Python examples using the API so below will show you how to get started with Go.
We start by making a simple Go file with a grpcSetup() method. Here we just find the users home directory and then create a path to the lnd directory.
Above we create a variable that holds our SSL/TLS credentials. The tls.cert file should have automatically been created for you when you ran the LND server for the first time. Printing creds should just show a memory address, don’t worry about this.
Above we create a gRPC header with our macaroons in it. These are security credentials that are meant to be passed with each call we make with the grpc API.
Above we create a connection to the gRPC LND server by specifying the host/port, and the credentials. We then return that connection from the grpcSetup() method and defer closing it.
Step 2: LNCLI setup and usage
Lets go through this line by line:
lncli := lnrpc.NewLightningClient(grpcConn)
This line creates a new Lightning Client that contains many methods that we can call. Here is the documentation on all the methods that can be called on this client.
ctx, _ := context.WithTimeout(context.Background(), 10*time.Second)
This line creates a context that will timeout after 10 seconds, not too important. However, this is required to be passed in to every method called from the Lightning Client.
walletBalanceReq := lnrpc.WalletBalanceRequest{}
This line creates the request that we will pass into the next function. It is empty because there is no information we need to provide in order to get our wallet balance.
walletRes, err := lncli.WalletBalance(ctx, &walletBalanceReq, MACAROONOPTION)
if err != nil { fmt.Println(err)}
These lines actually make the call to the Lightning Client to retrieve the wallet balance. As you can see we pass in the context, wallet balance request, and the macaroons that we created in the gRPC setup. If an error occurs, we will print it. If the LND server was not running when you ran this, you will probably see an error about not being able to find a connection.
fmt.Println(walletRes.TotalBalance)
Lastly, we print out the wallet balance to show that we actually retrieved something, it will most likely be 0 if you followed this tutorial from beginning to end.
Responses typically have many attributes you can view. Specifically, WalletBalance has attributes like TotalBalance, ConfirmedBalance, and UnconfirmedBalance. Click here to see the docs.
All together now
Here is the complete code that will get you started using the LND. Given this foundation, you should be able to build on-top of this and continue your LND journey.
If you’ve spotted any errors, feel free to contribute to the code here!
Learning Bitcoin’s Lightning Network with Go (Set up) was originally published in Hacker Noon on Medium, where people are continuing the conversation by highlighting and responding to this story.
Disclaimer
The views and opinions expressed in this article are solely those of the authors and do not reflect the views of Bitcoin Insider. Every investment and trading move involves risk - this is especially true for cryptocurrencies given their volatility. We strongly advise our readers to conduct their own research when making a decision.