Latest news about Bitcoin and all cryptocurrencies. Your daily crypto news habit.
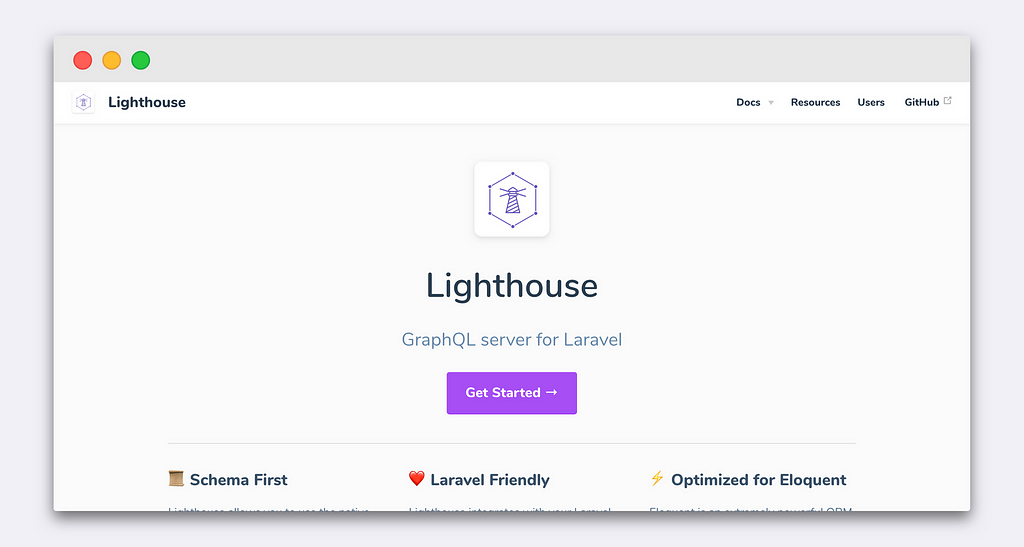
For a new project I’m working on, I had to code a GraphQL API with Laravel. I pulled in the Lighthouse GraphQL Server package, which was featured on Laravel News some weeks ago and started to follow the documentation. While most things worked as I expected, I spent quite a lot of time debugging some that didn’t and weren’t documented anywhere, so after I figured them out, I thought this article could help others facing the same position.
Password length checking
Imagine you have a createUser mutation to, uhhh, create users. It’s using the @bcrypt directive (provided by Lighthouse) to make our life easier: The code is as follows:
type Mutation { createUser( name: String @rules(apply: ["required"]) email: String @rules(apply: ["required", "email", "unique:users,email"]) password: String @rules(apply: ["required", "string", "min:6", "max:255"]) @bcrypt ): User @create(model: "App\\User")}
You’d expect a password with two characters to throw an error, but it actually works. Apparently, the client hashes the password and then performs validation. As a workaround, you can move the migration’s logic to a controller by executing php artisan lighthouse:mutation CreateUser and then manually hashing and creating a User.
<?phpnamespace App\Http\GraphQL\Mutations;
use App\User;use GraphQL\Type\Definition\ResolveInfo;use Nuwave\Lighthouse\Support\Contracts\GraphQLContext;
class CreateUser { public function resolve($rootValue, array $args, GraphQLContext $context = null, ResolveInfo $resolveInfo) { return User::create([ 'username' => $args['username'], 'email' => $args['email'], 'password' => bcrypt($args['password']), ]); } }
Policies
Lighthouse features a @can directive to check if the user's authorized to perform the specified action, but it only works for generic models (meaning you can check if the user has permissions to create models, but you can't check permissions like deletion against a singular model). The workaround for this is, again, move your mutation/query to a controlling, and check for permission there.
<?phpnamespace App\Http\GraphQL\Mutations;
use App\SecureResource;use GraphQL\Type\Definition\ResolveInfo;use Nuwave\Lighthouse\Support\Contracts\GraphQLContext;
class DoSecureStuff { public function resolve($rootValue, array $args, GraphQLContext $context = null, ResolveInfo $resolveInfo) { abort_unless(request()->user()->can('update', SecretRe
Disclaimer
The views and opinions expressed in this article are solely those of the authors and do not reflect the views of Bitcoin Insider. Every investment and trading move involves risk - this is especially true for cryptocurrencies given their volatility. We strongly advise our readers to conduct their own research when making a decision.